Create Dx Ball Game Using JavaScript
Creating a Dx Ball Game (a type of brick breaker game) in JavaScript involves setting up the game environment, creating the paddle, ball, and bricks, and handling collisions and animations. Here’s how you can create a simple version of the Dx Ball Game using HTML5 Canvas and JavaScript.
Step 1: Set Up the HTML Structure
Create an index.html
file to set up the game canvas.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>DX Ball Game</title>
<style>
canvas {
display: block;
margin: auto;
background: #000;
}
body {
text-align: center;
background: #000;
color: #fff;
font-family: Arial, sans-serif;
}
</style>
</head>
<body>
<h1>DX Ball Game</h1>
<canvas id="gameCanvas" width="800" height="600"></canvas>
<script src="game.js"></script>
</body>
</html>
You Might Like This
Step 2: Create the JavaScript File
Create a game.js
file to handle the game logic.
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
// Paddle properties
const paddleHeight = 10;
const paddleWidth = 75;
let paddleX = (canvas.width - paddleWidth) / 2;
const paddleSpeed = 7;
// Ball properties
const ballRadius = 10;
let x = canvas.width / 2;
let y = canvas.height - 30;
let dx = 2;
let dy = -2;
// Brick properties
const brickRowCount = 5;
const brickColumnCount = 9;
const brickWidth = 75;
const brickHeight = 20;
const brickPadding = 10;
const brickOffsetTop = 30;
const brickOffsetLeft = 30;
let bricks = [];
for (let c = 0; c < brickColumnCount; c++) {
bricks[c] = [];
for (let r = 0; r < brickRowCount; r++) {
bricks[c][r] = { x: 0, y: 0, status: 1 };
}
}
let rightPressed = false;
let leftPressed = false;
let score = 0;
let lives = 3;
document.addEventListener('keydown', keyDownHandler, false);
document.addEventListener('keyup', keyUpHandler, false);
function keyDownHandler(e) {
if (e.key === 'Right' || e.key === 'ArrowRight') {
rightPressed = true;
} else if (e.key === 'Left' || e.key === 'ArrowLeft') {
leftPressed = true;
}
}
function keyUpHandler(e) {
if (e.key === 'Right' || e.key === 'ArrowRight') {
rightPressed = false;
} else if (e.key === 'Left' || e.key === 'ArrowLeft') {
leftPressed = false;
}
}
function collisionDetection() {
for (let c = 0; c < brickColumnCount; c++) {
for (let r = 0; r < brickRowCount; r++) {
let b = bricks[c][r];
if (b.status === 1) {
if (
x > b.x &&
x < b.x + brickWidth &&
y > b.y &&
y < b.y + brickHeight
) {
dy = -dy;
b.status = 0;
score++;
if (score === brickRowCount * brickColumnCount) {
alert('YOU WIN, CONGRATULATIONS!');
document.location.reload();
}
}
}
}
}
}
function drawBall() {
ctx.beginPath();
ctx.arc(x, y, ballRadius, 0, Math.PI * 2);
ctx.fillStyle = '#0095DD';
ctx.fill();
ctx.closePath();
}
function drawPaddle() {
ctx.beginPath();
ctx.rect(paddleX, canvas.height - paddleHeight, paddleWidth, paddleHeight);
ctx.fillStyle = '#0095DD';
ctx.fill();
ctx.closePath();
}
function drawBricks() {
for (let c = 0; c < brickColumnCount; c++) {
for (let r = 0; r < brickRowCount; r++) {
if (bricks[c][r].status === 1) {
let brickX = c * (brickWidth + brickPadding) + brickOffsetLeft;
let brickY = r * (brickHeight + brickPadding) + brickOffsetTop;
bricks[c][r].x = brickX;
bricks[c][r].y = brickY;
ctx.beginPath();
ctx.rect(brickX, brickY, brickWidth, brickHeight);
ctx.fillStyle = '#0095DD';
ctx.fill();
ctx.closePath();
}
}
}
}
function drawScore() {
ctx.font = '16px Arial';
ctx.fillStyle = '#0095DD';
ctx.fillText('Score: ' + score, 8, 20);
}
function drawLives() {
ctx.font = '16px Arial';
ctx.fillStyle = '#0095DD';
ctx.fillText('Lives: ' + lives, canvas.width - 65, 20);
}
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBricks();
drawBall();
drawPaddle();
drawScore();
drawLives();
collisionDetection();
if (x + dx > canvas.width - ballRadius || x + dx < ballRadius) {
dx = -dx;
}
if (y + dy < ballRadius) {
dy = -dy;
} else if (y + dy > canvas.height - ballRadius) {
if (x > paddleX && x < paddleX + paddleWidth) {
dy = -dy;
} else {
lives--;
if (!lives) {
alert('GAME OVER');
document.location.reload();
} else {
x = canvas.width / 2;
y = canvas.height - 30;
dx = 2;
dy = -2;
paddleX = (canvas.width - paddleWidth) / 2;
}
}
}
if (rightPressed && paddleX < canvas.width - paddleWidth) {
paddleX += paddleSpeed;
} else if (leftPressed && paddleX > 0) {
paddleX -= paddleSpeed;
}
x += dx;
y += dy;
requestAnimationFrame(draw);
}
draw();
Game Mechanics
- Paddle Control:
- The player controls a paddle horizontally using the left and right arrow keys.
- The paddle is used to bounce the ball back into the field of bricks.
- Ball Movement:
- The ball moves continuously in a diagonal direction.
- When the ball hits a wall, it bounces off and continues in a different direction.
- The player must use the paddle to keep the ball in play and prevent it from falling off the bottom of the screen.
- Bricks:
- The game field is filled with rows and columns of bricks.
- Each time the ball hits a brick, the brick is destroyed, and the ball bounces off in a different direction.
- The goal is to destroy all the bricks to advance to the next level.
- Collision Detection:
- The game checks for collisions between the ball and the bricks, walls, and paddle.
- When a collision with a brick is detected, the brick is removed, and the ball changes direction.
- Score and Lives:
- The player earns points for each brick destroyed.
- The game displays the current score and the number of lives remaining.
- The player starts with a set number of lives, and each time the ball falls off the bottom of the screen, a life is lost.
- The game ends when all lives are lost.
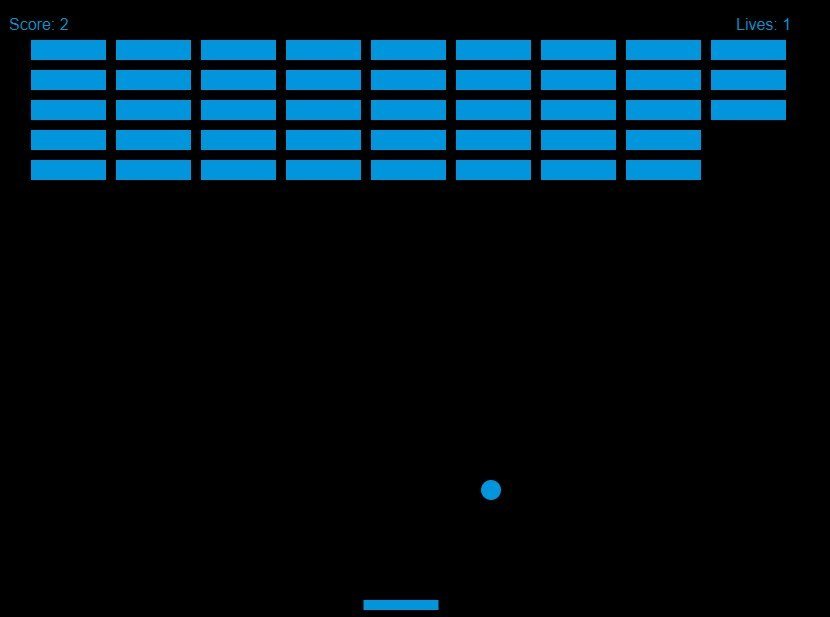
Explanation:
- HTML and CSS: The HTML sets up the canvas and styles for the game. The body is styled to have a black background with white text for better visibility.
- JavaScript:
- Canvas Setup: Initializes
canvas
andctx
for drawing. - Game Properties: Defines properties for the paddle, ball, bricks, score, and lives.
- Event Listeners: Listens for arrow key presses to move the paddle left and right.
- Collision Detection: Checks for collisions between the ball and bricks, and updates the score.
- Drawing Functions: Draws the ball, paddle, bricks, score, and lives on the canvas.
- Game Loop: The main game loop clears the canvas, draws all elements, updates the ball and paddle positions, checks for collisions, updates the score and lives, and repeats using
requestAnimationFrame
.
- Canvas Setup: Initializes
This example provides a basic DX Ball game that can be further enhanced with additional features like power-ups, multiple levels, sound effects, and advanced graphics.
Conclusion
DX Ball combines classic brick breaker mechanics with modern features to create an engaging and enjoyable gaming experience. With its simple yet challenging gameplay, vibrant graphics, and exciting power-ups, DX Ball provides endless hours of fun for players of all ages. Whether you’re aiming to beat high scores or just looking for a casual gaming experience, DX Ball offers a perfect blend of strategy and entertainment.