How to create form validation HTML & Js
Create a responsive form validation and hover effects using HTML and CSS, we can build a simple form structure with basic input validation and CSS styling. Here’s an example
Create index.html file and copy code and paste code our layout.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Form with Validation by Mycodingtutorial</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="form-container">
<form id="contactForm" action="#" method="POST" novalidate>
<h2>Contact Us</h2>
<div class="form-group">
<label for="name">Name</label>
<input type="text" id="name" name="name" placeholder="Enter your name" required>
<small>Error message</small>
</div>
<div class="form-group">
<label for="email">Email</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required>
<small>Error message</small>
</div>
<div class="form-group">
<label for="message">Message</label>
<textarea id="message" name="message" placeholder="Enter your message" required></textarea>
<small>Error message</small>
</div>
<button type="submit">Submit</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
Create style.css file and copy code and paste code our layout.
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.form-container {
width: 100%;
max-width: 500px;
background-color: white;
padding: 30px;
border-radius: 10px;
box-shadow: 0 4px 10px rgba(0, 0, 0, 0.1);
animation: fadeIn 0.5s ease-in-out; /* Animation for form container */
}
h2 {
text-align: center;
margin-bottom: 20px;
color: #333;
animation: slideDown 0.5s ease-in-out; /* Animation for heading */
}
.form-group {
margin-bottom: 20px;
opacity: 0;
transform: translateY(20px);
animation: fadeInUp 0.5s forwards ease-in-out; /* Input fields slide in with opacity */
}
.form-group:nth-child(2) {
animation-delay: 0.2s; /* Delay for Name input */
}
.form-group:nth-child(3) {
animation-delay: 0.4s; /* Delay for Email input */
}
.form-group:nth-child(4) {
animation-delay: 0.6s; /* Delay for Message input */
}
.form-group label {
display: block;
margin-bottom: 8px;
color: #555;
}
.form-group input, .form-group textarea {
width: 100%;
padding: 10px;
border: 2px solid #ddd;
border-radius: 5px;
font-size: 16px;
outline: none;
transition: border-color 0.3s ease-in-out, transform 0.3s ease-in-out;
}
.form-group input:hover, .form-group textarea:hover {
border-color: #007bff;
}
.form-group input:focus, .form-group textarea:focus {
border-color: #007bff;
transform: scale(1.02); /* Slight zoom on focus */
}
small {
color: red;
visibility: hidden;
margin-top: 5px;
display: block;
opacity: 0;
transition: opacity 0.5s ease-in-out; /* Fade effect for error messages */
}
button {
width: 100%;
padding: 10px;
background-color: #007bff;
border: none;
color: white;
font-size: 16px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease-in-out, transform 0.3s ease-in-out;
animation: fadeIn 0.5s ease-in-out; /* Button fade-in animation */
animation-delay: 0.8s; /* Delay to match other animations */
}
button:hover {
background-color: #0056b3;
transform: scale(1.05); /* Button zooms in on hover */
}
/* Responsive design */
@media screen and (max-width: 768px) {
.form-container {
padding: 20px;
}
button {
font-size: 14px;
}
}
/* Animations */
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
@keyframes slideDown {
from {
transform: translateY(-30px);
opacity: 0;
}
to {
transform: translateY(0);
opacity: 1;
}
}
@keyframes fadeInUp {
from {
opacity: 0;
transform: translateY(20px);
}
to {
opacity: 1;
transform: translateY(0);
}
}
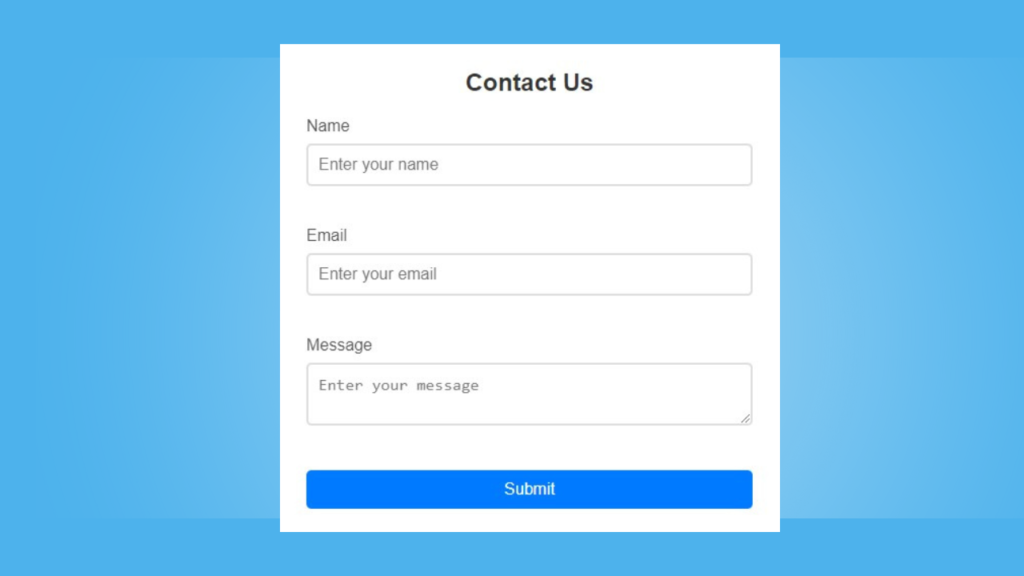
Create script.js file and copy code and paste code our layout.
const form = document.getElementById('contactForm');
const nameInput = document.getElementById('name');
const emailInput = document.getElementById('email');
const messageInput = document.getElementById('message');
form.addEventListener('submit', (e) => {
e.preventDefault();
validateInputs();
});
function validateInputs() {
// Validate Name
const nameValue = nameInput.value.trim();
if (nameValue === '') {
setError(nameInput, 'Name cannot be empty');
} else {
setSuccess(nameInput);
}
// Validate Email
const emailValue = emailInput.value.trim();
if (emailValue === '') {
setError(emailInput, 'Email cannot be empty');
} else if (!isValidEmail(emailValue)) {
setError(emailInput, 'Not a valid email');
} else {
setSuccess(emailInput);
}
// Validate Message
const messageValue = messageInput.value.trim();
if (messageValue === '') {
setError(messageInput, 'Message cannot be empty');
} else {
setSuccess(messageInput);
}
}
function setError(input, message) {
const formGroup = input.parentElement;
const small = formGroup.querySelector('small');
small.textContent = message;
small.style.visibility = 'visible';
small.style.opacity = '1';
input.style.borderColor = 'red';
}
function setSuccess(input) {
const formGroup = input.parentElement;
const small = formGroup.querySelector('small');
small.style.visibility = 'hidden';
small.style.opacity = '0';
input.style.borderColor = '#007bff';
}
function isValidEmail(email) {
const re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@(([^<>()[\]\.,;:\s@"]+\.)+[^<>()[\]\.,;:\s@"]{2,})$/i;
return re.test(String(email).toLowerCase());
}
Key Features
- Responsive Design:
- The form adjusts to screen sizes with the
@media
queries, making it fully responsive on both desktop and mobile.
- The form adjusts to screen sizes with the
- Hover Effects:
- Input fields and the submit button have hover effects using
:hover
in CSS to change the border and background colors when users hover over them.
- Input fields and the submit button have hover effects using
- Validation:
- JavaScript is used for form validation:
- The
validateInputs
function checks if the name, email, and message fields are empty. - Email validation uses a regex to check if the email format is correct.
- If an input is invalid, an error message is shown below the input field using the
<small>
tag, and the input border turns red. If valid, the input border turns blue.
- The
- JavaScript is used for form validation:
- Mobile-friendly:
- The form looks great on all devices due to its responsive nature. It shrinks and adjusts the layout for mobile screens using media queries.
This form can be easily extended with additional fields, improved styling, or server-side validation for production use.